Introduction to C Language Syntax
C is a general-purpose programming language that has been around since the 1970s. It is known for its efficiency and control, and it’s the basis for many modern programming languages.
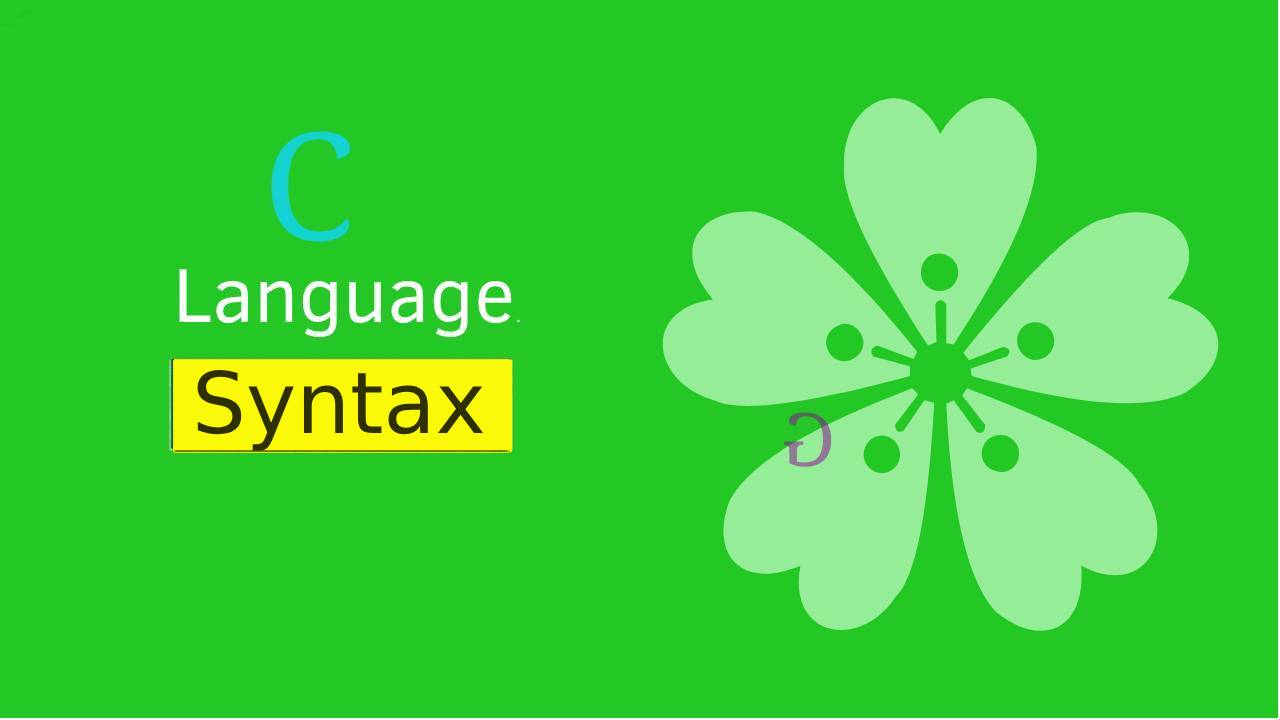
Basic Structure of a C Program
A C program typically includes the following components:
- Preprocessor Commands: These are directives for the C preprocessor. They usually start with a
#
symbol, such as#include <stdio.h>
. - Functions: A function is a group of statements that perform a specific task. Every C program has at least one function, which is
main()
. The execution of a C programming language syntax starts from themain()
function. - Variables: Variables are used to store data. In C, every variable has a specific type, such as
int
,char
, orfloat
. - Statements & Expressions: Statements represent actions or commands. Expressions are combinations of variables, constants and operators written according to the syntax of the language.
- Comments: Comments are used to explain sections of code. In C, comments can be single-line (
//
) or multi-line (/*...*/
).
Here’s an example of a simple C program:
#include <stdio.h>
int main() {
// printf() displays the string inside quotation
printf("Hello, World!");
return 0;
}
Data Types in C
C supports several different types of data, each of which has unique properties. These include:
- Integer Types: These include
char
,int
, andshort
. - Floating-Point Types: These include
float
anddouble
. - Void Type: The
void
type represents the absence of value.
Control Structures in C
Control structures dictate the flow of control in a program. These include:
- Sequential Structures: Code is executed sequentially by default.
- Selection Structures: These include
if
,if...else
, andswitch
statements. - Loop Structures: These include
for
,while
, anddo...while
loops.
Conclusion
Understanding the syntax of C is crucial for mastering the language. With its clear structure and powerful capabilities, C continues to be a popular choice for programmers around the world.