C language introduction:
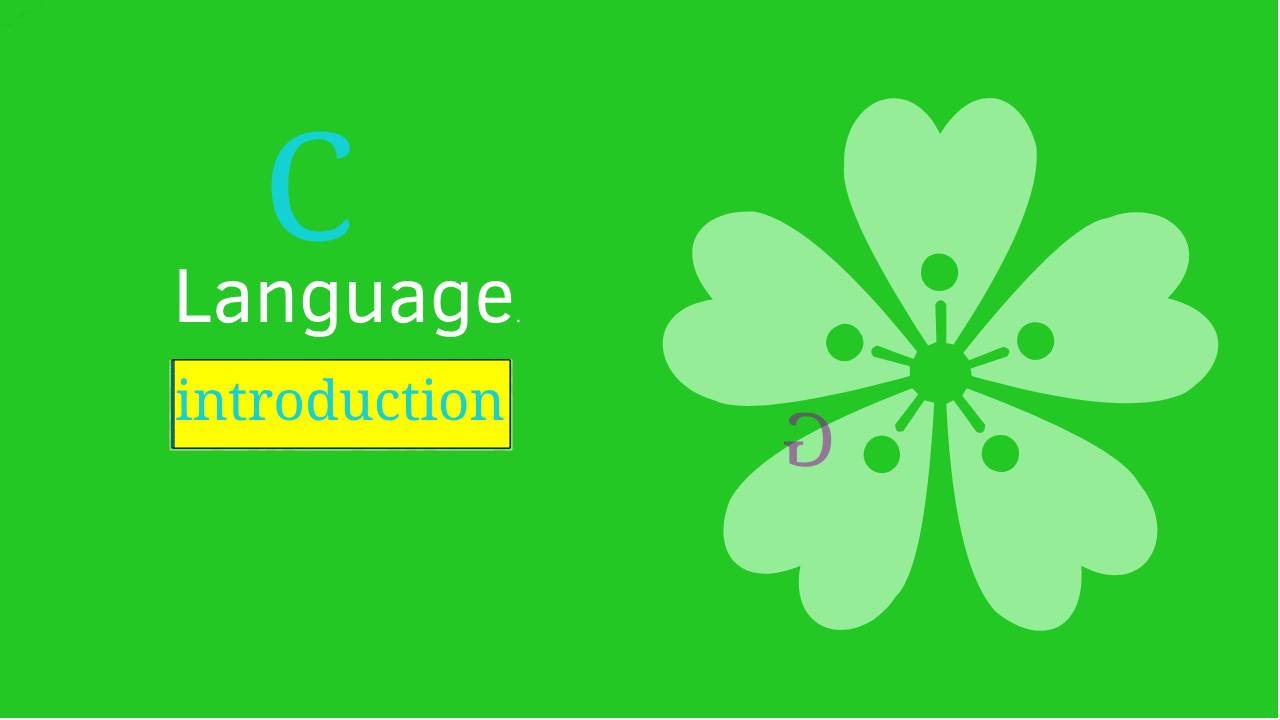
C is one of the most widely used programming languages in the world. It was developed by Dennis Ritchie at Bell Labs in the early 1970s as a system programming language for Unix operating systems. C is a powerful, efficient, and portable language that supports low-level access to memory, structured programming, and recursion. C is also the basis for many other popular languages such as C++, Java, Python, and C#.
In this blog post, we will learn about some of the basic features and syntax of C language. We will also see some examples of how to write and run a simple C program.
Features of C Language
Some of the main features of C language are:
- Procedural Language: C is a procedural language, which means that it follows a step-by-step approach to solve a problem. A C program consists of one or more functions that perform specific tasks. The main function is the starting point of the program execution.
- Fast and Efficient: C is a compiled language, which means that the source code is converted into executable code by a compiler before running. This makes C faster and more efficient than interpreted languages like Python or Ruby.
- Modularity: C supports modularity, which means that the code can be divided into separate files and libraries for reuse and maintenance. The #include directive allows us to include other files or libraries in our program.
- Statically Typed: C is a statically typed language, which means that the type of each variable is checked at compile time. This helps to avoid errors and improve performance. We have to declare the type of each variable before using it in our program.
- General-Purpose Language: C can be used for various applications such as operating systems, databases, compilers, games, embedded systems, etc. C has a rich set of built-in operators and functions that can manipulate data and perform calculations.
- Middle-Level Language: C is a middle-level language, which means that it combines the features of both low-level and high-level languages. C allows us to access and manipulate the hardware directly using pointers and bitwise operators. C also provides high-level features such as structures, unions, enums, etc.
Syntax of C Language
The syntax of C language is the set of rules that govern how we write and structure our code. The basic syntax of a C program consists of:
- Header Files: These are files that contain declarations and definitions of functions, variables, macros, etc. that we can use in our program. We use the #include directive to include header files in our program. For example, #include <stdio.h> includes the standard input/output library that provides functions like printf() and scanf().
- Main Function: This is the function where the execution of the program begins. Every C program must have one and only one main function. The main function has the following syntax:
int main()
{
// statements
return 0;
}
The int keyword indicates that the main function returns an integer value. The return 0 statement indicates that the program ends successfully. The curly braces {} enclose the body of the function where we write our statements.
- Statements: These are instructions that tell the computer what to do. Each statement must end with a semicolon (;). For example, printf(“Hello World!\n”); is a statement that prints “Hello World!” on the screen.
- Comments: These are lines that are ignored by the compiler and are used to explain or document the code. Comments can be either single-line or multi-line. Single-line comments start with // and end at the end of the line. Multi-line comments start with /* and end with */. For example:
// This is a single-line comment
/* This is
a multi-line
comment */
Example of a Simple C Program
Let us see an example of how to write and run a simple C program that prints “Hello World!” on the screen.
// Include the standard input/output library
#include <stdio.h>
// Define the main function
int main()
{
// Print "Hello World!" on the screen
printf("Hello World!\n");
// Return 0 to indicate successful termination
return 0;
}
To run this program, we need to save it in a file with .c extension, such as hello.c. Then we need to compile it using a C compiler such as gcc or clang. For example, on Linux or Mac OS X, we can use the following command in a terminal:
gcc hello.c -o hello
This will create an executable file named hello in the same directory as hello.c. To run this file, we can use the following command:
./hello
This will produce the following output:
Hello World!
Congratulations! You have just written and run your first C program.
Conclusion
In this blog post, we have learned about some of the basic features and syntax of C language. We have also seen how to write and run a simple C program. C is a powerful and versatile language that can be used for various applications. If you want to learn more about C language, you can check out some of the following resources: